Google Play Services Tutorial For Unity
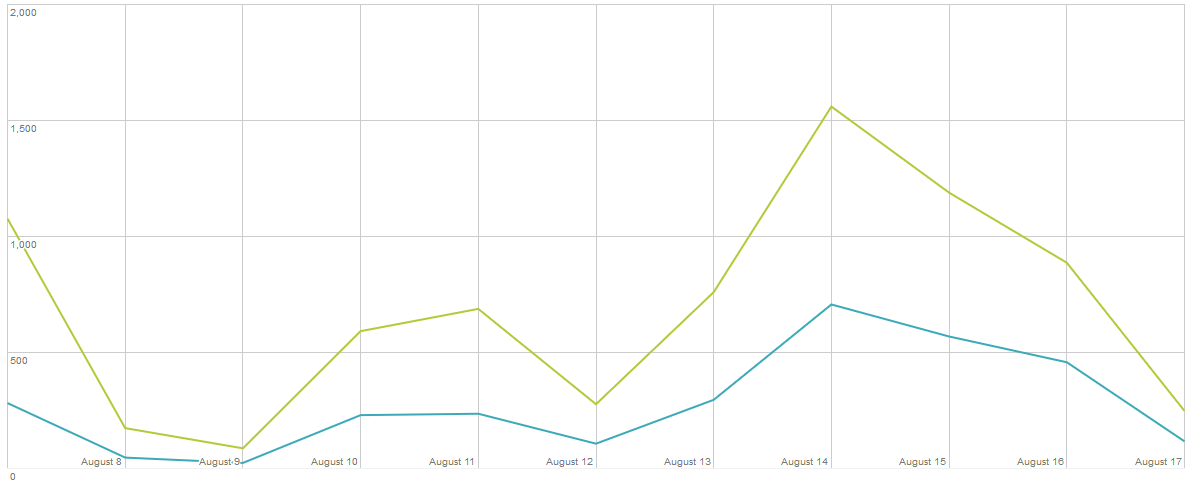
Recently I have been putting the finishing touches on my next game and naturally that includes implementing Google Play Services into the app. Achievements, leaderboards, and other stats are a great way to keep your players engaged in the game and to keep things interesting. Luckily the process is pretty simple and straightforward with Unity, and Google maintains a great plugin that makes this very easy. However, I’ll provide a few quick tips and guidelines on how to get your implementation up and running quickly.
The first thing you need to do is create your project/app in the Google Developer Console. You will want to setup the app & register to use play services before you begin. You can add some achievements and a leader board, as well as any other features you might want to include.
Next you will import the play services plugin into Unity. Get the latest build from the Github repository, open your unity project, and just run the unitypackage you just downloaded to import all the files into your project. You’ll now be able to setup your project by navigating to Window->Google Play Games->Setup->Android setup.
From here, you need to provide two things, the constants class name and resources definition. I’ll recommend you name the constants class name the same as your app, and for the resources definition, grab it from the Google Play Developer Console, in either the achievements or leaderboard section.
Now that the basics are setup, you’ll need to write a few scripts to actually use the services in your game.
The first thing you’ll want to do is to make the user sign in.
using UnityEngine; using System.Collections; using GooglePlayGames; using UnityEngine.SocialPlatforms; public class GooglePlayLogin : MonoBehaviour { Â Â Â void Awake() Â Â Â { Â Â Â Â Â Â Â Social.localUser.Authenticate((bool success) => { Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â if (success) Â Â Â Â Â Â Â Â Â Â Â { Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â PlayGamesPlatform.Activate(); Â Â Â Â Â Â Â Â Â Â Â } Â Â Â Â Â Â Â }); Â Â Â } }
Social.localUser.Authenticate will attempt to sign-in, if successful, then we want to activate the play services platform. When we call Activate(), this will make Google Play Services the default implementation for unity social platform calls. You’ll need this script running when the game launches, so put it on a game object that will exist on launch. If you test now, you’ll see a prompt to sign-in or a successful toast if successful.
Note that you’ll only be able to use these services while testing on your android device. You won’t be able to test anything using the Unity Remote nor the editor player.
Achievements
Keeping track of your players achievement progress is easy. I would recommend implementing your own achievements system in addition to using Play Services so that your players who don’t use Google Play can also unlock them.
Social.ReportProgress(achievementID, 100f, (bool success) =>
{
//handle callback
});
To unlock / complete an achievement, call ReportProgress with a value of 100, with 0 being locked. However, to report incremental progress, Google recommends using the following instead of the Unity implementation:
           PlayGamesPlatform.Instance.IncrementAchievement(achievementID, incrementAmount, (bool success) =>            {                //handle callback            });
With this, you can increment your achievement progress as a progressive unlock.
Leaderboards
Leaderboards are a great way to keep players engaged and returning for more. The sense of competition is great and it’s always nice to see how you stack up against the other players. For developers such as yourself, it’s a great tool to see how players are doing in your game.
Social.ReportScore(score, leaderboardID, (bool success) =>            {                //handle callback            });
Events
I really like using events as you can obtain so much player data just from simple inputs. You can track everything from how often a player starts a new round, presses a certain button, or how often they die to a certain enemy and find an item. I’m not entirely sure of how robust this method is for tracking large amounts of different data, but for gathering many of your basic data it is a great tool. I’m able to use this player data to figure out which parts of the game my players are having trouble with, which levels they enjoy, and even discover bugs.
I typically track items such as:
- Times a level is loaded
- Completion rate of a level
- Player Accuracy
- Enemies Killed
- Abilities Used
I really wish to know which parts of my game players are playing. It helps me improve the game as well as give hints to what parts of the game need improving.
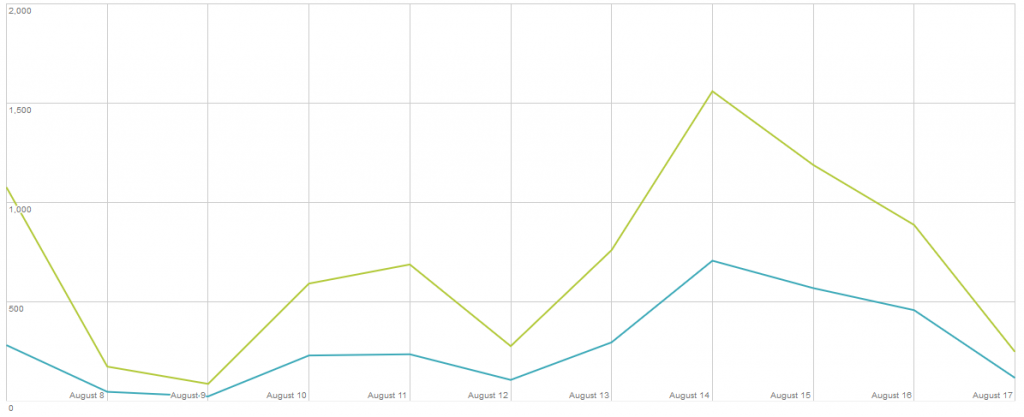
Just like the other Google Play features, events are rather easy to use as well. To increment an event:
PlayGamesPlatform.Instance.Events.IncrementEvent(eventName, incrementAmount);
Since the Unity Social implementation doesn’t support events, you will need to call the IncrementEvent method of PlayGamesPlatform.
Platform Compatibility and Tips
The Android implementation is rather simple. It is possible to implement Play Services on iOS as well, however you will also need to install Cocoapods. I would recommend you stick to Game Center for iOS to avoid the extra dependencies. To disable Play Services for iOS, you must add NO_GPGS to “Scripting Define Symbols” which can be found in Edit -> Project Settings -> Player -> iPhone -> Other Settings -> Scripting Define Symbols.
I would also recommend implementing your own achievement, leaderboard, and event methods. This way you can layer your functions over Google Play / Game Center without any code conflicts. By creating your own “Services Manager” you can decouple your services from Unity as well as independent platforms. Take advantage of platform directives so that one method can run code for multiple platforms.
Sample of how to use them:
#if UNITY_IPHONE
Debug.Log("Iphone");
#elif UNITY_ANDROID
Debug.Log("Android");
#endif
Hope this tutorial helps. These features are a great addition to any mobile game and there’s no real downside to implementing them. Let me know your results and be sure to check out the plugin github for extensive information on all of the available features.
Interested in learning more about Unity? Check my other Unity tutorials.
Jonathan
Hey there, I’m Jon! I love landscape photography which is why I travel all the time! On my blog I’ll share all the best spots for epic sunsets and sunrises as well as some photography tips and tricks. I also have a interest in game design so you’ll find the occasional post about that too. Thanks for visiting!