AI-Powered Unity Game Development with GitHub Copilot: Long Term Review
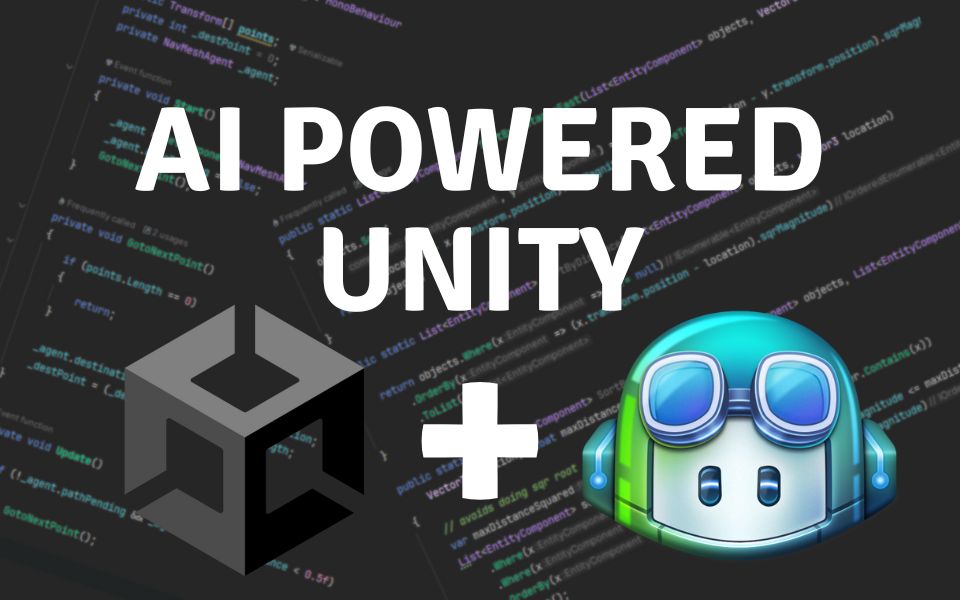
As a Unity developer, you know that creating great games and experiences takes a lot of hard work and dedication. But what if there was a way to make the coding process a little bit easier and more efficient? Well, there is and it’s called GitHub Copilot!
GitHub Copilot is an AI-powered coding assistant that can help you write code faster and more accurately. It can generate boilerplate code, suggest relevant code snippets, and even provide context-aware suggestions. In other words, it’s like having a coding buddy who’s always there to help you out.
I’ve been using GitHub Copilot with Unity for the past six months, and I’m here to tell you that it’s a game-changer. It’s been a great help with coding and has increase my productivity.
Note: This review has been updated to reflect my long-term usage of GitHub Copilot for Unity development (past the initial 6 month period of the original review). Copilot Chat is finally public so I added a new section that talks entirely about chat with examples on how I use it.
Getting GitHub Copilot
Copilot is available for all the popular IDEs used for Unity (Visual Studio, VS Code, and Jetbrains Rider). It requires a subscription ($10/month or $100/year), however there is a free month long trial you can sign up for to evaluate the service.
Once you get your subscription setup, install the GitHub copilot plugin in the IDE of your choice. After you enable the plugin it should start working right away.
Suggestions will start to appear as you code and by default tab
is used to accept these suggestions. I still like to keep my IDE-provided settings though so I rebound my apply completions
key for copilot to shift + tab
.
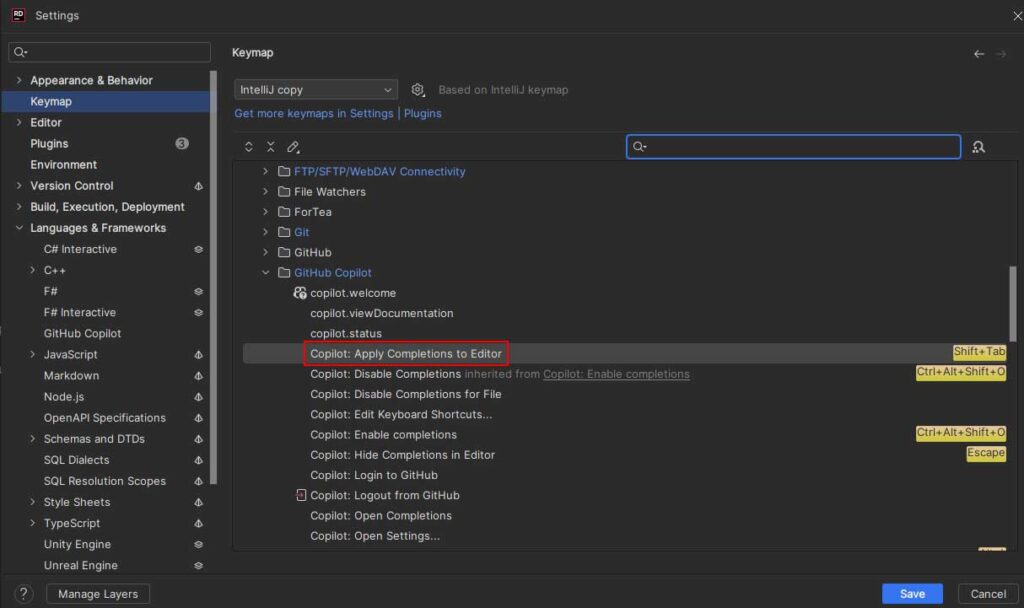
The Power of GitHub Copilot in Unity Development
1. Boilerplate Code Generation
GitHub Copilot is great at generating boilerplate code for Unity games. Whether it’s setting up components/monobehaviours, creating UI code, or defining game logic, Copilot can usually anticipate what I’m looking for and generate the code I need.
Even in situations where it doesn’t suggest the correct solution it usually generates a solution that is “close enough” to what I’m looking for where I can make a few changes to get it working.
Here’s an example of what copilot produced when asking for a patrol component. No edits were made to the code.
using UnityEngine;
using UnityEngine.AI;
// Monobehavior that handles patrolling for an enemy between points and uses nav
// mesh agent to control the movement
public class PatrollingComponent : MonoBehaviour {
public Transform[] points;
private int _destPoint = 0;
private NavMeshAgent _agent;
private void Start() {
_agent = GetComponent<NavMeshAgent>();
_agent.autoBraking = false;
GotoNextPoint();
}
private void GotoNextPoint() {
if (points.Length == 0) {
return;
}
_agent.destination = points[_destPoint].position;
_destPoint = (_destPoint + 1) % points.Length;
}
private void Update() {
if (!_agent.pathPending && _agent.remainingDistance < 0.5f) {
GotoNextPoint();
}
}
private void OnDrawGizmos() {
if (points.Length == 0) {
return;
}
Gizmos.color = Color.red;
foreach (var point in points) {
Gizmos.DrawSphere(point.position, 0.1f);
}
}
}
2. Intelligent Auto-Completion
One of my favorite features of Copilot is it’s context aware auto completion. As I write code, Copilot suggests entire lines or blocks based on context, making it feel like having a coding partner right next to you. This is great when working with library specific APIs such as Unity Physics or networking APIs as I don’t always remember all the APIs. Instead of searching online for documentation copilot usually gives enough of a hint that I remember what methods I wanted to call or how I should implement certain logic.
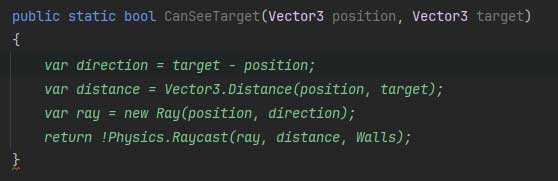
Sometimes it’s a bit too enthusiastic with the completion suggestions though so I still keep the normal IDE suggestions on. This is why I set the keybind for Copilot completions on a different keybind (Shift + Tab)
3. Copilot Chat Inside Your IDE!
Copilot Chat is like having a stronger Chat GPT window inside your IDE. Instead of having to copy and paste code you can directly reference your code files and chat with Copilot. Ask it to refactor your code, design questions, or even general Unity questions.
One of the biggest improvements to Copilot is the ability for it to automatically reference your existing files in your code base. This provides much better context to the AI when generating relevant code.
In the following example, I asked Copilot to help me create a component that spawns game objects within a rectangle are at a random interval. I specifically ask it to use FloatRange
which is a helper class I have in my project that allows you to define a min/max and get a random value from it. I expect it to expose a game object and FloatRange field in the component so it can be customized in the inspector. Here is the output:
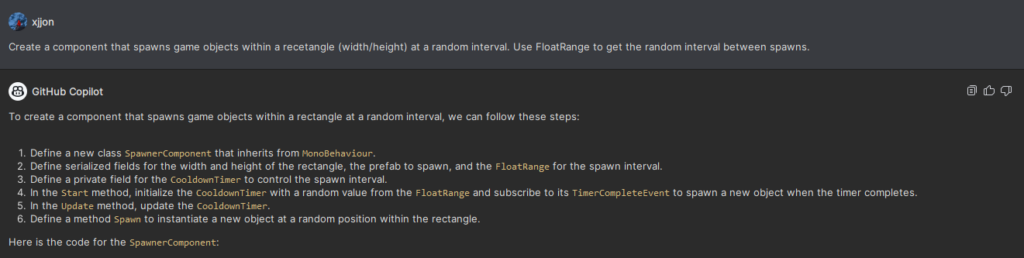
using UnityEngine;
using Util;
using Util.Range;
namespace Core.Components
{
public class SpawnerComponent : MonoBehaviour
{
[SerializeField] private GameObject _prefab;
[SerializeField] private float _width = 10f;
[SerializeField] private float _height = 10f;
[SerializeField] private FloatRange _spawnInterval = new FloatRange(1f, 5f);
private CooldownTimer _spawnTimer;
void Start()
{
_spawnTimer = new CooldownTimer(_spawnInterval.Random());
_spawnTimer.TimerCompleteEvent += Spawn;
_spawnTimer.Start();
}
void Update()
{
_spawnTimer.Update(Time.deltaTime);
}
private void Spawn()
{
Vector3 spawnPosition = new Vector3(
transform.position.x + Random.Range(-_width / 2, _width / 2),
transform.position.y,
transform.position.z + Random.Range(-_height / 2, _height / 2)
);
Instantiate(_prefab, spawnPosition, Quaternion.identity);
_spawnTimer.Start(_spawnInterval.Random());
}
}
}
The code output is almost exactly as expected. It created the right fields and even used CooldownTimer
which is another helper class I have in my project to wrap duration based events. This shows that it has context of other files in my project, as I didn’t directly reference any existing code with this prompt.
At the end of the prompt it shows what files it referenced to generate this code. It even uses the same code style as my other files, which is how it also picks up on code patterns such as using CooldownTimer
.

Let’s try one more example and ask Copilot to improve the Spawn() method by using my object pool instead of creating a new instance each time. As you can see, it referenced `PrefabPool` which is my custom object pool and used it to get an instance of the prefab!
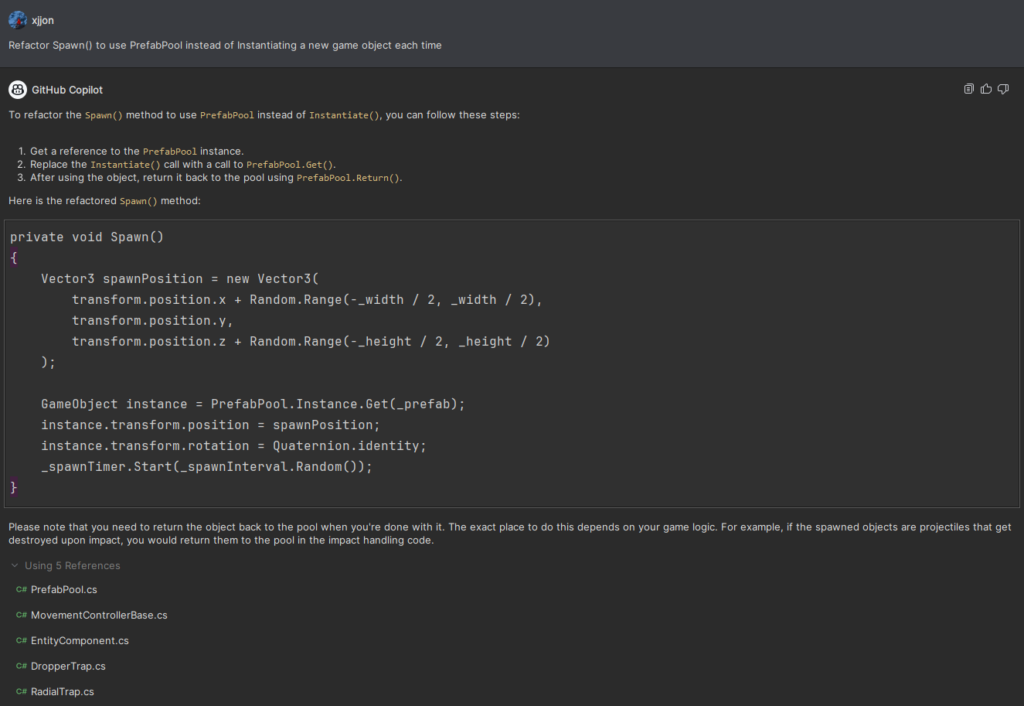
Real-world Results: Boosted Unity Productivity
1. Time Savings
The most tangible benefit I’ve experienced is the significant time savings. I’m able to write code much quicker and this efficiency has allowed me to focus on the creative aspects of game development rather than getting bogged down by repetitive coding tasks.
It takes a little bit to get used to having Copilot with you when you code but once you get the hang of it you almost are able to “predict” what suggestions it’s going to give you and tab-complete your way to success.
2. Code Quality
There’s a lot of concern about the quality of auto-generated code and those concerns are mostly valid. Especially for less experienced programmers, auto-generated code can be of poor quality and sometimes even dangerous. However, I’ve found that if you review the code it gives you and make changes where needed, it can still produce high quality code.
Treat your AI-powered Copilot as a tool and not a source of truth and you should be fine. It’s similar to looking for solutions on stackoverflow or the Unity forums – you still should validate and verify the code and not just blindly copy and paste things into your project.
Another area Copilot has been great in is generating Unit Tests. It generally writes pretty good tests, even for MonoBehaviours and has definitely increased the code coverage of my games.
Recommendations for Unity Developers
After almost a year of integrating GitHub Copilot into my Unity development workflow, I highly recommend this tool to all Unity developers. I think GitHub Copilot can boost your productivity in Unity regardless of your level of experience. It’s constantly improving and getting smarter as the team at GitHub adds new features. Here are a few more tips for maximizing its effectiveness:
- Explore Copilot Suggestions: Don’t just use Copilot for routine tasks; explore its suggestions for more complex logic and algorithms. You might be surprised at the innovative solutions it proposes.
- Provide Context: While Copilot is impressive, it benefits from clear context. Writing clear and concise comments or method names helps Copilot generate more accurate and relevant code snippets. Use the chat! Manually reference your files if it’s not able to pick up on what you are asking.
I’ll continue to update this review as I use Copilot. GitHub is continuously adding new features to Copilot and it’ll be interesting to see how this AI powered coding assistant evolves over time.
Interested in more? Check out my other Unity content or see what my favorite Unity tools are.
Jonathan
Hey there, I’m Jon! I love landscape photography which is why I travel all the time! On my blog I’ll share all the best spots for epic sunsets and sunrises as well as some photography tips and tricks. I also have a interest in game design so you’ll find the occasional post about that too. Thanks for visiting!