Creating A Pause In Unity
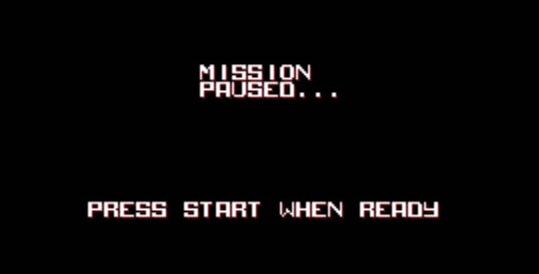
Creating a pause function in Unity is veryeasy to implement. It only takes a few simple lines of code yet it’s a commonly asked question, so I’ll show you a quick and easy way to implement pausing of your game in Unity.
Time.timeScale = 0f;
Time.timeScale is the scale of which time passes by in your application. Set it to 0 and any functions that are time dependent will stop. To un-pause, just do the opposite.
Time.timeScale = 1.0f;
Note that everything that is time dependent will stop, so any animations will stop moving as well. However, it’s likely that your pause menu and UI need to continue to animate even after the game is paused. You can fix this by setting those animations to update using Unscaled Time.
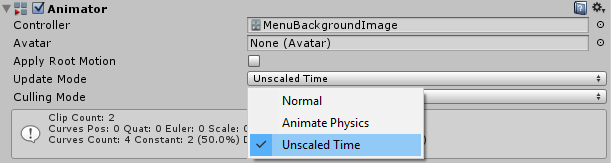
This is found in the Animator component of your game object. In this example, it’s for my main menu. I like my menus to drop down from the top of the screen when the game is paused, so they need to stay moving.
Here’s a simple snippet you can use to pause your game that saves the state in a boolean. The function toggles between paused and unpaused states, so you can use the same function throughout different parts of your game.
public bool isPaused;
/**
* Toggles game pause and returns the game state afterward.
**/
public bool Pause()
{
if (isPaused)
{
isPaused = false;
Time.timeScale = 1.0f;
}
else
{
isPaused = true;
Time.timeScale = 0f;
}
return isPaused;
}
Another useful way to use the isPaused boolean is to set it as a condition when getting player input. It’s likely you don’t want to player to be able to move the camera or character around when the game is paused, so you’ll need to stop that.
void Update()
{
if (isPaused) return;Â Â Â Â //returns if game is paused
}
Note that FixedUpdate isn’t called when timeScale is set to 0
Anyways, that’s all there is to this simple method of pausing your game in Unity. Just remember that Update() isn’t affected by timeScale, so it’s still called as per normal. Thanks for reading and happy coding!
Bonus: It might be a little finicky, but Time.timeScale can be used to speedup / slowdown time within your game. Since everything affected by time will be scaled by it, you could use it to create some slow-motion and speed-boosts as well. It affects your whole game though, so you may want to implement this using your own multiplier variable.
Interested in more Unity tutorials? Click here!
Jonathan
Hey there, I’m Jon! I love landscape photography which is why I travel all the time! On my blog I’ll share all the best spots for epic sunsets and sunrises as well as some photography tips and tricks. I also have a interest in game design so you’ll find the occasional post about that too. Thanks for visiting!
Thanks! This is very helpful!