Easy Unity Ads & Mediation for Mobile Tutorial
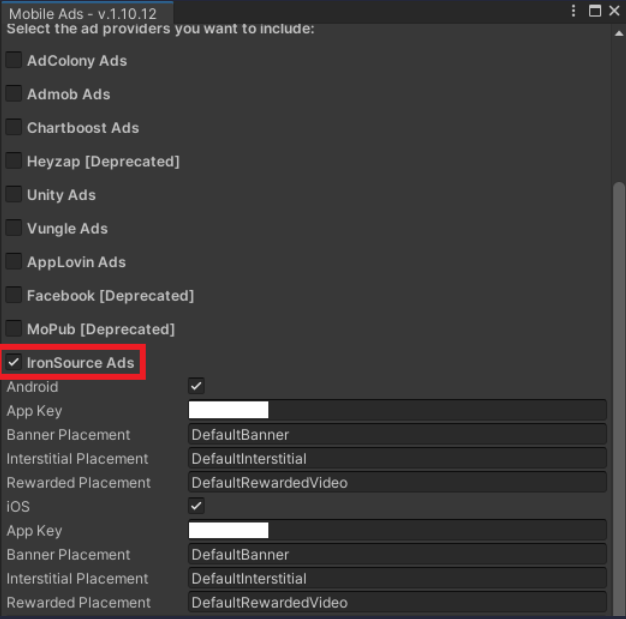
Monetizing your mobile game is essential in generating revenue from your game and in this tutorial I will show you the easiest way I’ve found to integrate Ads & Mediation in Unity. We will take advantage of a really good asset to speed up our development and save time. We will also use Unity LevelPlay for Ad Mediation to maximize our revenue through network bidding. This ensures that the highest paying Ad is displayed to your gamers at all times.
Summary
- Setup mediation and ad networks to boost your game revenue
- Use the Unity Mobile Tools asset to quickly integrate with different Ad SDKs
- Load and display rewarded video ads within your game
Estimated Time: 1 Hour
Step 1: Required Assets
The asset that we’ll be using is called Mobile Tools by Gley. It contains many useful features for mobile games such as ads, notifications, in app purchases (IAPs), etc. I highly recommend the bundle to save yourself time as it’s time consuming to integrate each of these features for both Android and iOS. If you solely require ad functionality, Gley also offers a more affordable standalone Mobile Ads package for $40.

Step 2: Set Up Ad Network Accounts
Before diving into the integration process, setup/create accounts with ad providers/networks. I recommend using Ironsource, Unity, and Google AdMob. I found that these networks offer good availability but also the best payouts (eCPM). Let me know in the comments below if you find better options!
You’ll need to setup your app within each of the 3 providers and then setup a default ad unit as well. I recommend using rewarded interstitial (video) ads as they tend to have the best payouts and are least annoying to users.
Once you have them setup, add the app info and ad unit info to Ironsource (as this is what we will use for mediation). This is done in Ironsource on the Setup -> SDK Networks page.

Step 3: Enable Ad Mediation with Unity LevelPlay
- Download the Unity LevelPlay package and import it into your Unity project.
- Obtain relevant adapters for the ad networks you intend to use. You can find these in the “Ads Mediation” -> “Integration Manager” within Unity LevelPlay.
I use Unity Ads and AdMob (ironsource is enabled by default).
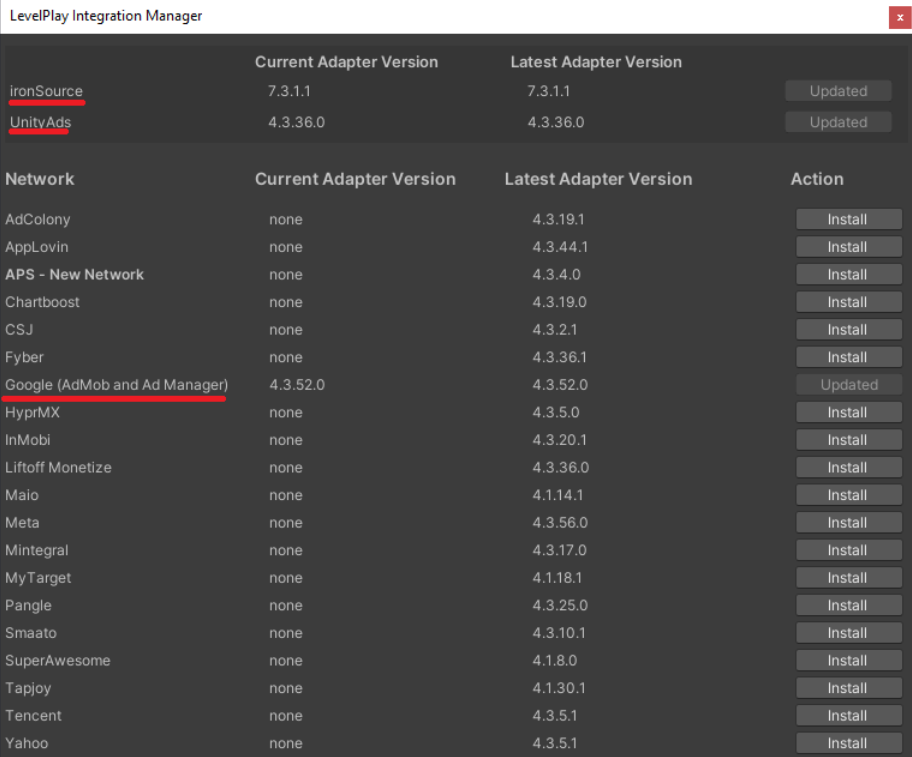
Next enable Ironsource integration through the Mobile Toolkit plugin (Window -> Gley -> Mobile Ads). Note we keep Admob and Unity Ads disabled since we will serve the ads for all 3 networks via Ad Mediation (LevelPlay).
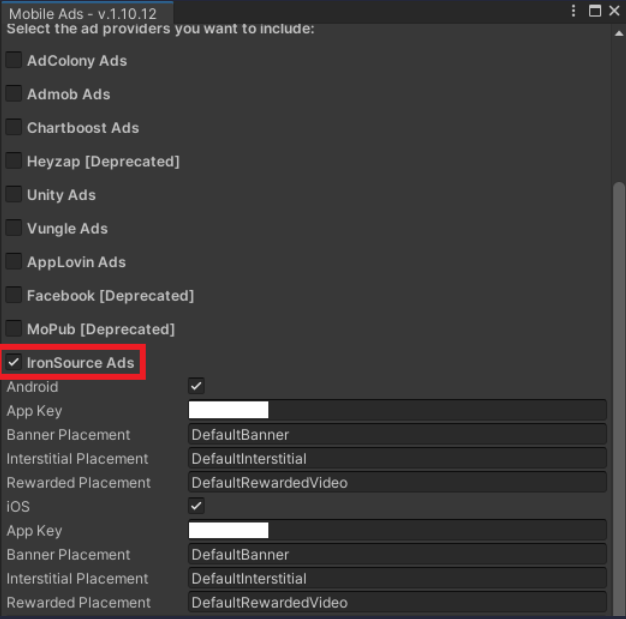
Step 4: Initializing Ads SDKs
Next, let’s initialize the Gley Ads implementation. Create a monobehaviour with an Awake() function in your main scene and add the following code:
using UnityEngine;
public class AdManager : MonoBehaviour
{
void Awake()
{
Advertisements.Instance.Initialize();
}
}
This component could be a singleton with DoNotDestroyOnLoad() so that your game only has one instance of it.
Step 5: Show Rewarded Video Ads
With the Gley Ads API, displaying rewarded video ads is straightforward. Use the following code snippet to show rewarded videos:
Advertisements.Instance.ShowRewardedVideo((adCompletionStatus) =>
{
// Implement code based on whether the ad was completed or not
});
Step 6: Test Your Build
Do a test android and iOS build to make sure everything compiles correctly. You can enable debug mode from the Mobile Tools plugin settings to see in-game diagnostics on which ad networks are loaded. They will also be available through your device logs in xcode or ADB.
Note: If you get this error:
Dependency ':androidx.core.core-1.9.0:' requires 'compileSdkVersion' to be set to 33 or higher.
Compilation target for module ':launcher' is 'android-32'
To fix, set your target API level in build settings to at least android-32 or above.
Conclusion
Here is a full example of how I load and display Ads in my games. It includes some sample integration code on how to send analytics to Firebase so you can track how often players skip ads, encounter errors, or complete an ad view.
public bool ShowRewardAd(AdLocation location, UnityAction<bool> onComplete) {
if (!IsRewardAdReady(location)) {
return false;
}
try {
Advertisements.Instance.ShowRewardedVideo((status) => {
ReportAdCompletionStatus(location, status);
onComplete?.Invoke(status);
});
if (FirebaseManager.Initialized) {
FirebaseAnalytics.LogEvent("AdStarted",
FirebaseAnalytics.ParameterAdUnitName,
location.ToString());
}
} catch (Exception e) {
Debug.Log("Failed to show ad: " + e.Message);
// Show prompt to user that ad failed to load
if (FirebaseManager.Initialized) {
Crashlytics.Log(
$"Rewarded Ad Failed for {location} with {e.Message}");
FirebaseAnalytics.LogEvent("AdFailed",
FirebaseAnalytics.ParameterAdUnitName,
location.ToString());
}
return false;
}
return true;
}
It wraps the Gley Advertisements
API to perform some additional error handling and analytics reporting. Use the AdLocation
enum to specify all the parts of your game that might display ads.
Congratulations! You have now learned how to easily integrate ads into your Unity game using the Mobile Tools asset by Gley, along with implementing ad mediation with Unity LevelPlay (Ironsource). I hope these steps work for you and enable you monetize your game successfully! Happy game development!
Looking for more assets to help with your Unity game development? Check out my post on the Best Unity assets and how they can supercharge your productivity!
Jonathan
Hey there, I’m Jon! I love landscape photography which is why I travel all the time! On my blog I’ll share all the best spots for epic sunsets and sunrises as well as some photography tips and tricks. I also have a interest in game design so you’ll find the occasional post about that too. Thanks for visiting!
This is great. It used to take so long to setup ads!