Unity Cooldown Timer Script Tutorial
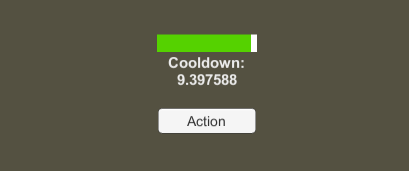
I wrote a tutorial a few years ago for creating a cooldown timer in unity. While that version worked fine, I’ve decided to update the tutorial and script with a more robust version that better suits unity. This cooldown timer is designed to be used for anything from abilities, monster spawners, weapon attacks, and much more.
Difficulty: Easy – You should know the basics of Unity and be comfortable writing small amounts of C# code.
Github: Get it here.
Code Setup
For the purpose of a fully working tutorial and demo, I included some unity UI elements and other scripts to help you visualize the cooldown timer. Those parts of code are unnecessary and you can remove them. All you need is the code contained within CooldownTimer.cs
Before we dive into the code, let’s go over some of the details with this unity cooldown timer implementation.
The timer follows the Unity Update
pattern. This is implemented through void Update(float timeDelta)
. The reason is this allows the timer to be controlled via Unity MonoBehaviours. The timer should be updated via a MonoBehaviour that is active in the scene. This has the added benefit of following unity time (which means you can pause the timers by using Time.timeScale).
A TimerCompleteEvent
is exposed by the cooldown timer. This event should be used to trigger whatever action needs to be completed when the timer is complete (spawn monster, cast ability, etc).
CooldownTimer.cs
public class CooldownTimer
{
public float TimeRemaining { get; private set; }
public float TotalTime { get; private set; }
public bool IsRecurring { get; }
public bool IsActive { get; private set; }
public int TimesCounted { get; private set; }
public float TimeElapsed => TotalTime - TimeRemaining;
public float PercentElapsed => TimeElapsed / TotalTime;
public bool IsCompleted => TimeRemaining <= 0;
public delegate void TimerCompleteHandler();
public event TimerCompleteHandler TimerCompleteEvent;
public CooldownTimer(float time, bool recurring = false)
{
TotalTime = time;
IsRecurring = recurring;
TimeRemaining = TotalTime;
}
public void Start()
{
if (IsActive) { TimesCounted++; }
TimeRemaining = TotalTime;
IsActive = true;
if (TimeRemaining <= 0)
{
TimerCompleteEvent?.Invoke();
}
}
public void Start(float time)
{
TotalTime = time;
Start();
}
public void Update(float timeDelta)
{
if (TimeRemaining > 0 && IsActive)
{
TimeRemaining -= timeDelta;
if (TimeRemaining <= 0)
{
if (IsRecurring)
{
TimeRemaining = TotalTime;
}
else
{
IsActive = false;
}
TimerCompleteEvent?.Invoke();
TimesCounted++;
}
}
}
public void Invoke()
{
TimerCompleteEvent?.Invoke();
}
public void Pause()
{
IsActive = false;
}
public void AddTime(float time)
{
TimeRemaining += time;
TotalTime += time;
}
}
UI Demo Setup
The next part looks at the demo setup and basic usage of the cooldown timer. If you want to test it out you can pull the project and then press “Start” button to begin the timer. When the timer is complete, it will trigger an event that sets the text to say “Cooldown Completed!”
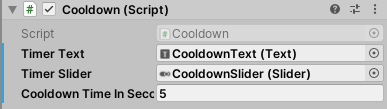
The demo contains a GameObject Action1
that contains the Cooldown script and associated UI elements. The canvas contains a UI Slider and a UI Text which shows the % remaining as well as the time remaining.
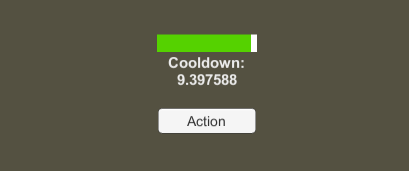
How to use the cooldown timer in Unity
The CooldownTimer exposes a event called TimerCompleteEvent
. The easiest way to use the timer is to register a handler that responds to the timer event.
You can do that by:
Defining a method
void SetupTimer() {
_cooldownTimer.TimerCompleteEvent += OnTimerComplete;
}
void OnTimerComplete() {
//Do Something
}
Defining an anonymous handler
void SetupTimer() {
_cooldownTimer.TimerCompleteEvent += () => { //do something };
}
The best way to do it would be through a method. This allows you to un-register the handler if you need to.
// un-register the handler
_cooldownTimer.TimerCompleteEvent -= OnTimerComplete;
Update the timer
Lastly, you need to call CooldownTimer.Update(float time) to actually update the timer. Usually you would do this in a monobehaviour Update() function.
void Update() {
_cooldownTimer.Update(Time.deltaTime);
}
Additional Tips and Examples
CooldownTimer.cs
exposes a few helper fields such as TimeElapsed, PercentElapsed, PercentRemaining, and IsCompleted. These provide an easy way to get the state of the cooldown timer.
CooldownTimer.Start(float time)
can be called with a different start time to start the cooldown timer with a new time. This is helpful if you want to create something with variable times (such as a monster spawner). You can do that by doing something like this:
_cooldownTimer.Start(Random.Range(minimumSpawnTime, maximumSpawnTime));
The CooldownTimer can be used to prevent abilities or attacks to be completed until after the cooldown is completed. A simple way to do that would be:
public void Attack() {
if (_cooldownTimer.IsActive)
{
return;
}
_cooldownTimer.Start();
// Do attack logic
}
Conclusion
So there’s the updated cooldown tutorial for Unity. It should be a lot easier to setup and use across your projects now. Feel free to grab the code from github. If you have a use case not covered by the examples, let me know! I’ll be happy to help.
Interested in learning more about Unity? Check out my other unity tutorials!
If you have any questions, drop a comment below or send me a message.
Jonathan
Hey there, I’m Jon! I love landscape photography which is why I travel all the time! On my blog I’ll share all the best spots for epic sunsets and sunrises as well as some photography tips and tricks. I also have a interest in game design so you’ll find the occasional post about that too. Thanks for visiting!