Simple State Machines
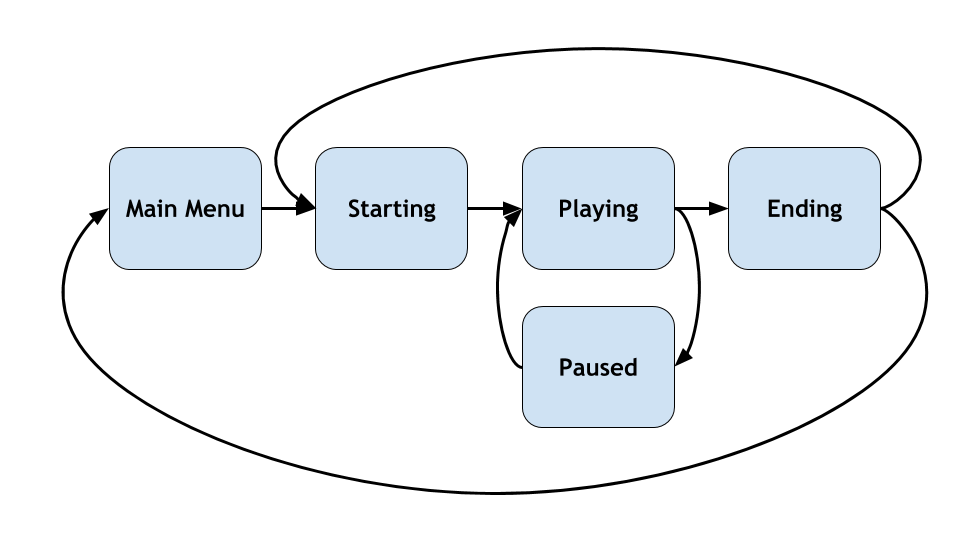
Quite commonly game state machines are designed to be overly complex and hard to understand. As the game expands, they become larger and harder to follow. Somehow the game still magically works and most of the time nothing is done to simplify it. Generally most games revolve around a few states and limiting the number of states makes the game more maintainable.
What Are State Machines?
A state machine is a mathematical model of a number of finite states that can only be in exactly one state at any given time. Transitions between states are predefined and can only take place along those paths. Put more simply, a game can only be in a few states at any given time. For example, the game is either playing, paused, restarting, or ended. It’s not possible to be in multiple states at any given time, and the transitions between each state are clear cut.
Essentially the parts of the state machine are:
- A number of finite states that correspond to a certain behavior
- Transitions between one state to another
- Rules that trigger transitions
Simple State Machines
So how can we design a simple, yet functional state machine? Start with the basics. Every game should at the bare minimum contain a playing state, a starting state when the game is loading, and an ending state. There will possibly a pause state and maybe a menu state. Here’s what it would look like:
The arrows represent transitions between each state. There are rules that correspond to when the transitions can be triggered. For example, pressing pause would change the game from playing to paused. When the game is ending, it can either go into the main menu or restart back to starting.
Implementing in Unity
As the title goes, it’s actually pretty simple to implement this kind of design in Unity.
To start, we need an interface to build all our states with.
public interface IGameState { Â Â Â public IGameState Update(float timeDelta); }
public class PlayingState : IGameState {    public IGameState Update(float timeDelta){        //game logic        return this;    } }
Jonathan
Hey there, I’m Jon! I love landscape photography which is why I travel all the time! On my blog I’ll share all the best spots for epic sunsets and sunrises as well as some photography tips and tricks. I also have a interest in game design so you’ll find the occasional post about that too. Thanks for visiting!