Simple JSON to Store Game Data in Unity
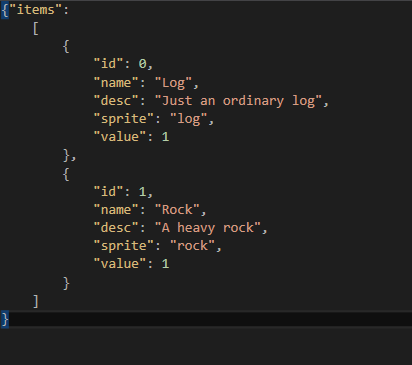
In this Unity tutorial, I’ll show you how to effortlessly read JSON files to import data into your game. JSON is an excellent way to manage your game data and seamlessly load it as Unity objects during runtime. Games typically involve a lot of data that used for different game mechanics and behaviors. Examples include items and abilities, each with their unique attributes like attack damage, defense, health, item value, and more. We’ll explore different methods to address this data management challenge.
Some common approaches to handle game data in Unity:
- Hard Coded Data: Beginners or someone prototyping may be more inclined towards this, but generally you want to stay away from hard coding any data into your code.
- Prefabs and Inspector: Creating prefabs and editing values for each prefab may work for smaller games, but could you imagine managing thousands of items through the inspector?
- Scriptable Objects: Slightly better than prefabs, you’re still editing data through the inspector though, which is time confusing and not the easiest. Still a big problem when it comes to being scale-able.
There must be a better solution right? For anyone who’s sifted through large number of prefabs to make small changes, I’ve got something to make your life easier. This is where storing data in JSON format makes things way easier. JSON is a human-readable way to format data that utilizes attribute:pair values to store data. It’s easy to read, easy to understand, easy to use, and very flexible.
Using JSON with your Unity Objects
Let’s say you wanted to store item data for your game. Each item might have an ID, name, description, sprite, and perhaps a sell value. A class representation of this item would look something like this.
[Serializable]
public class Item {
  Â
   public int Id;
   public string Name;
   public string Description;
   public string Sprite;
   public float Value;
  Â
}
If this was a MonoBehavior, you would be able to add it as a component, then create prefabs for each item. Works when you only have a couple items, but once again fails to scale very well. So let’s look at how a JSON representation of a couple items would look like.
{"items":
   [
       {
           "id": 0,
           "name": "Log",
           "desc": "Just an ordinary log",
           "sprite": "log",
           "value": 1
       },
       {
           "id": 1,
           "name": "Rock",
           "desc": "A heavy rock",
           "sprite": "rock",
           "value": 1
       }
   ]
}
Here we have two items, a Log and a Rock. Each item has it’s own values and it’s easy to understand. It’s much more efficient to be editing your data this way than through an inspector. You can even use third party JSON editors or input your data in Excel and convert it to JSON later on.
So now we have our item data in JSON format, but what about actually getting it to work with Unity? Luckily Unity provides easy to use utility methods to serialize and deserialize JSON objects.
We will be loading our JSON data from the Resources folder of the project. If you don’t have one, you can create the folder inside the Assets folder. It’s actually quite simple to load the file, but before we do so, we need to create a wrapper to handle an array of JSON elements.
Building upon our Item class from before, let’s create a new class ItemArray to wrap around the Items.
[Serializable]
class ItemArray
{
   public Item[] items;
}
Reading the JSON objects
Now when we deserialize our JSON data, we will receive an array of Items. On to the actual reading of the JSON file. (My file is named items.json).
TextAsset file = Resources.Load("items") as TextAsset;
  Â
ItemArray items = JsonUtility.FromJson<ItemArray>(file.text);
      Â
foreach (var item in items.items)
{
    Debug.Log(item);
}
It’s actually just two lines. One to load the JSON file from the Resources folder and another line to parse the JSON data into our Item objects. The last two just print out all the Items from the ItemArray, which should represent your JSON data in object form.
So there you have it, a simple way to read data from JSON into your Unity game. You’ll now be able to store all your game data in easy to read and edit JSON files and avoid dealing with the difficulties of prefabs and scriptable objects. In future tutorials I’ll demonstrate how you can use this data to dynamically create your game objects too, so that all your game data and information can be stored externally. Of course you can store your data in other popular formats as well, such as XML, CVS, or even a database such as SQL or MongoDB. Hope this tutorial has been helpful and easy to understand!
Interested in learning more about Unity? Check out my other Unity tutorials!
Jonathan
Hey there, I’m Jon! I love landscape photography which is why I travel all the time! On my blog I’ll share all the best spots for epic sunsets and sunrises as well as some photography tips and tricks. I also have a interest in game design so you’ll find the occasional post about that too. Thanks for visiting!
Would be wonderul if you posted a link to the project demo, or if you could do the follow up. Have a school project that needs this, and havent been able to find the resource.
Which part are you having trouble with? What do you mean by resource?
Here is a small gist that includes all the imports: https://gist.github.com/xjjon/2bf90deae852f777783faca081f94ed1